Basic skills in R: Working with data structures
Vector
What is a vector in R? You can store more than just one value inside a variable. You can, for example, make a variable called "ages_of_friends" and store a row of numeric values inside this variable. A variable that holds a row of values of the same data type, is called a vector. You can make numerical, character and logical vectors.
Creating a vector in R
Explanation
You create a vector with the function c()
(c is short for combine or concatenate). Within the parentheses you list the values that you want in your vector, separated by a comma.
Don't forget to give a name to your new variable and to enter a sequence of basic objects there. With no argument, you only specify that you are creating a vector object Look at the example to see how you could it.
There are also special instructions to create specific vectors. For example, v1 <- 2:4
creates the vector \((2,3,4)\)v2 <- seq(from=3, to=10, by=2)
creates the vector \((3,5,7,9)\).
In simulations one make extensive use of vectors with randomly generated numbers, i.e., with random numbers. There are several instructions for this purpose.
runif() |
random numbers between 0 and 1 using a uniform distribution |
sample() |
objects randomly taken from a vector |
rnorm() |
random numbers using a normal distribution |
Sample script
# create a numerical vector\(\phantom{x)}\)
num_vector <- c(20, 19, 21, 19)
print(num_vector)
# output: 20, 19, 21, 19
# create a character vector
char_vector <- c("Skye", "Leopold", "Jemma")
print(char_vector)
# output: "Skye" "Leopold" "Jemma"
# create a logical vector
logical_vector <- c(T, F, T, T)
print(logical_vector)
# output: TRUE FALSE TRUE TRUE
# create a vector of 3 randomly genenerated
# numbers between 0 and 1runif(3, min=0, max=1)
# output: this could look like
#[1] 0.4422001 0.7989248 0.1218993
# 3 random numbers via standard normal distriution
rnorm(3)
# output: this could look like
#[1] -0.4721671 1.7665132 0.8769968
Arithmetic with vectors
Explanation
Just as with variables with only one value stored in them, you can do math with vectors. When you add, subtract, multiply, or divide vectors, R will do the calculation for components at the same position. See the first example tot the right
But what if you want to add different numbers to each component of your vector? For example, you have the vector v1 <- c(1,2,3)
and want to add 2 to the first component, 6 to the second and 4 to the third component. You can do this by creating another vector and adding this vector to your original vector. Take a look at the second example to the right to get an idea.
Sample script
# Arithmetics with one numeric vector\(\phantom{x}\)
# create one numeric vector
v1 <- c(1, 2, 3)
# add 5 to the components of the vector
v1 + 5
#output: 6, 7, 8
# multiply the vector by 2
print(v1*2)
#output: 2, 4, 6
# Arithmetics with two vectors
# Create two numeric vectors
v1 <- c(1, 2, 3)
v2 <- c(4, 5, 6)
# add these vectors
added_vectors <- v1 + v2
print(added_vectors)
# output: 5 7 9
# multiply the vectors componentwise
multiplied_vectors <- v1 * v2
print(multiplied_vectors)
# output: 4 10 18
Selecting components of vectors and assigning values
Explanation
Vectors are subscriptable and mutable objects. This means that components of a vector (also called elements) can be accessed via a positional index and that you can change a vector also by assigning a value to a single component. Indexing starts at 1 and that there smart methods to create some vectors. Look at the first sample session to the right for selection of components.
You may want to ask R to give you all values in a vector that meet a certain condition. For example, you want to see all values in a vector that are greater than a particular value. You do that by telling R the name of the vector and including the condition you want the values to meet within square brackets.
In fact you use then logical indexing (with values TRUE and FALSE) to select components of vectors. You need relational operators for this, such as the ones in the table below.
== |
equal to |
< |
less than |
> |
greater than |
<= |
less than or equal to |
>= |
greater than or equal to |
!= |
not equal to |
You can also combine multiple logical expressions. Logical operators are listed below.
& or && |
and |
| or || |
or |
xor() |
exclusive or |
! |
not (logical negation) |
The second interactive sample session to the right shows an example of a selection of components of a vector via logical expressions.
Components to which you refer can also be assigned new values. By in-line assignments you cannot change the structure of the vector. See the third interactive sample session to the right to discover how you can assign components of a vector.
Sample sessions
Session 1
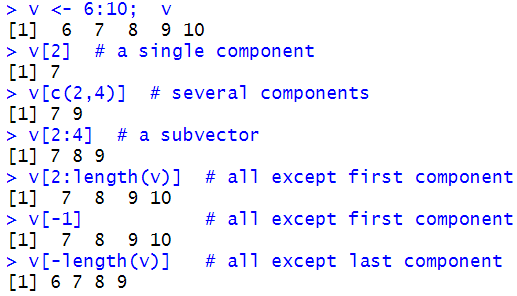
Session 2
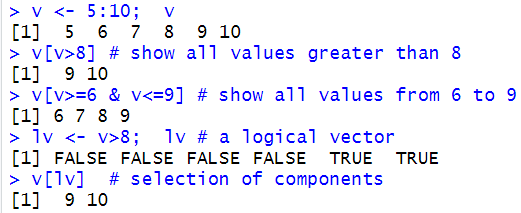
Session 3
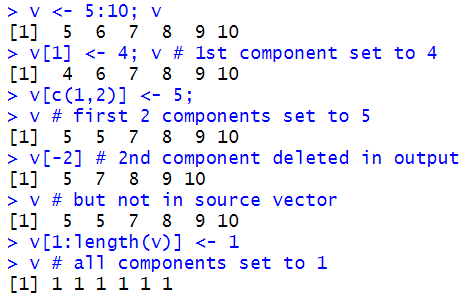
Ordering components of a vector
Explanation
To sort the values from lowest to highest value you can use the function sort()
. To sort from highest to lowest, you can either include the decreasing = TRUE
option when using the function sort()
, or first sort the vector using sort()
and then reverse the sorted vector using the function rev()
.
Perhaps a more useful task would be to sort one vector according to the values of another vector. To do this you use the function order()
in combination with selection via []
.
See the interactive sample sessions to the right.
Sample sessions
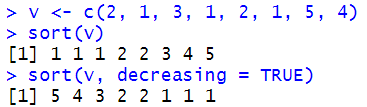
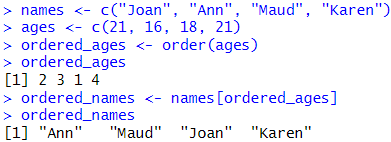