Basic skills in R: Working with data structures
List
What is a list in R? In the picture below you see data structures treated so far plus one more, namely a list. Recall that
- a vector is a finite sequence of objects of the same data type;
- a matrix is a two-dimensional object with elements in rows and columns of the same data type;
- a data frame is a two-dimensional object with elements in rows and columns, but colums may differ in data type;
- an array is a multi-dimensional object with elements of the same data type.
In a matrix, a data frame, or an array, all sequences in a particular dimension have the same length. What is missing is a data structure in which you can gather a variety of (possibly unrelated) objects under one name. This data structure in R is called a list. For example, a list may contain a combination of vectors, matrices, data frames, and even other lists.
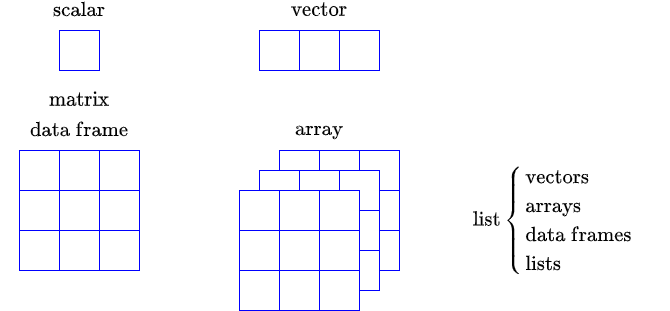
Working with lists in R
Explanation
You create a list with the function list()
. Between the parentheses you put the object that you want to store in your list, separated by comma's.
You can name elements of a list so that you can refer to a name in order to select a list element.
Selection of a list eleiment is doen via double square brackets [[...]]
The sample session on the right-hand side exemplifies working with lists
Sample session
> most_read_books <- c("The Bible",
+ "The Holy Quran") > largest_country_populations <-
+ c(1413, 1399, 335) # in millions > magic_square <- matrix(c(4,3,8,9,5,1,
+ 2,7,6), nrow=3) > my_list <- list(
+ books = most_read_books, + largest_country_populations,
+ magic_square) > my_list $books [1] "The Bible" "The Holy Quran" [[2]] [1] 1413 1399 335 [[3]] [,1] [,2] [,3] [1,] 4 9 2 [2,] 3 5 7 [3,] 8 1 6 > my_list[[3]] # select 3rd list element [,1] [,2] [,3] [1,] 4 9 2 [2,] 3 5 7 [3,] 8 1 6
> my_list[["books"]] # select 1st list element [1] "The Bible" "The Holy Quran"
> my_list$books [1] "The Bible" "The Holy Quran"
> # select 3rd component of 2nd list element
> my_list[[c(2,3)]] [1] 335