In the interactive R session below, there is a warning first because the sqrt
function only calculates within the non-negative real numbers.
> sqrt(-3)
[1] NaN
Warning message:
In sqrt(-3) : NaNs produced
If you want to be able to calculate square roots without any worries, you must be able to distinguish between negative and non-negative numbers. You can use a conditional statement via if
for this:
Onderstaand R script
x <- -3
if (x<0) {
cat("There is no real root of", x)
} else {
cat("sqrt(",x,") =", sqrt(x))
}
levert bij uitvoeren van de instructies de volgende tekst op
There is no real root of -3
\(\phantom{x}\)
The general form of a conditional statement ( if statement) is below. Read the explanation:
if (condition) {
indented instructions in case the condition is met
} else {
indented instructions in case the condition is not met
}
Two of the above lines are indented (starting with four spaces in this case): one after the if
and one after the else
. This means that precisely those lines are under the control of the if
and the else
, respectively. We've written indented instructions... here to indicate that there may be one or more R statements spread over multiple lines. We call the part containing the statements that will be executed if the condition is met the then part; the part that contains the statements that will be executed if the condition is not met is called the else part. So which part of the statements will be executed depends on the value of the condition during code execution: if the condition evaluates TRUE
, the statements in the then part are executed and the execution continues after the conditional statement; otherwise (i.e., with a value FALSE
) the statements in the else part are executed. The else part can also be omitted and then the output just continues where the conditional statement ends
The flowchart below shows the possible execution of the conditional command.
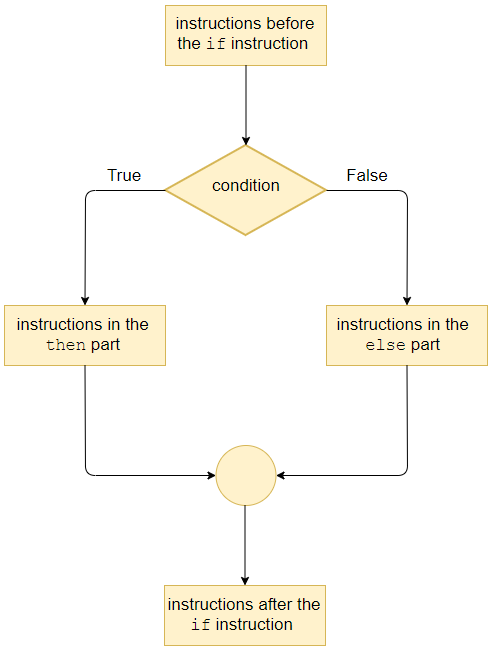
In our first example, we gave a variable a value that depended on a condition. This is so common that an inline ifelse
conditional statement is also possible in R. We could also have used the following R statement in the first example:
> root <- ifelse(x<0, "no number", sqrt(x))
We give another example session in R of the two kinds of conditional instructions, where the calculation depends on whether an integer is even or odd. The conditional instruction is spread over several lines, and the plus signs indicate that the instruction is not yet complete.
> x <- 5
> if (x %% 2 == 0) {
+ x <- x %/% 2
+ } else {
+ x <- 3*x + 1 + }
> x
[1] 16
> x <- ifelse(x %% 2 == 0, x %/% 2, 3*x + 1)
> x [1] 8
Finally, we give some examples of chained and nested conditional instructions. Take a good look at them, try to understand them and read the explanations of the examples.
If you run the following R script
numbers_NL <- c("een", "twee", "drie")
choice <- sample(numbers_NL, 1)
cat(choice, "\n")
if (choice == "een") {
cat("one\n")
} else if (choice == "twee") {
cat("two\n")
} else if (choice == "drie") {
cat("three\n")
} else {
cat("???\n")
}
switch(choice,
"een" = cat("one\n"),
"twee" = cat("two\n"),
"drie" = cat("three\n")
)
this may yield the following output (depending on the actual choice made):
The sample
function allows us to select a random element from a vector of three Dutch number names.
After this, there is a chain of conditional instructions.
In this conditional branch, only one choice is ultimately made. The last branch is missing the else part. In an unchained conditional statement, this corresponds to the following flowchart:
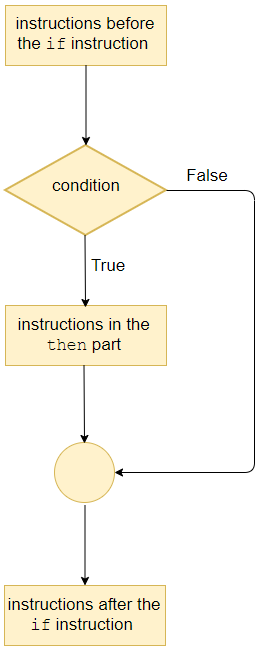
Because the nested conditions can get a bit cluttered, even if the else if
construct is used, there is also a switch
construct in R to distinguish between different cases.
For the example below, read the explanation for information about formatting printed numbers.
If you run the following R script
r <- runif(1) # a random number between 0 and 1
io <- sprintf("r = %.2f\n", r) # formatted text
cat(io)
if (0.25<r) {
if (r<0.75) {
io <- sprintf("%.2f is a number between 0.25 and 0.75\n", r)
}
}
cat(io)
this can produce the following output:
r = 0.39
0.39 is a number between 0.25 and 0.75
0.39 is a number between 0.25 and 0.75
With the runif(1)
function we generate a random floating-point number between 0 and 1 using the uniform distribution. By calling an if statement within an if statement, also called nested use of conditional statements, we can check whether the drawn number is in the segment \([0.25, 0.75]\) and then print a statement about it. But two short, more understandable abbreviations are possible. You can combine conditions with a logical operator. In this example, we use the &&
operator to test whether two conditions are met.
In this example, we also introduced a formatted output example:
creates a string where the given floating-point number is printed with 2 digits after the decimal point.
The table below summarises the most commonly used formatting specifications.
\[\begin{array}{l|c|l}
\textit{Type } & \textit{Specificatio} & \textit{Explanation} \\ \hline
\text{integer} & \mathtt{\%}w\mathbf{d} & \text{The integer is placed in a textfield of width }w\text{.}\\[-2pt]
& & w \text{ may be left out.}\\ \hline
\text{double} & \mathtt{\%}w.d\mathbf{f} & \text{The floating-point number is placed in a textfield of width }w\\[-2pt]
& & \text{(including the decimal poind) with } d \text{ decimals}\\[-2pt]
& & w\text{ and }d\text{ may be left out.}\\ \hline
\text{double} & \mathtt{\%}w.d\mathbf{e} & \text{is placed in a textfield of width }w\\[-2pt]
&\mathtt{\%}w.d\mathbf{E} & \text{(including the decimal point) with } d \text{ decimals in scientific notation.}\\[-2pt]
& & w\text{ and }d\text{ may be left out.}\\ \hline
\text{numeric} & \mathtt{\%}\mathbf{g} \text{ or } \mathtt{\%}\mathbf{g} & \text{Compact decimal notation or scientific notation.}\\ \hline
\text{character} & \mathtt{\%}w\mathbf{s} & \text{The string is placed in a textfield of width }w\text{.}
\end{array}\]
Besides the newline escape character \n
there are more escape characters. The table below summarises the most commonly used escape characters.
\[\begin{array}{c|l}
\textit{Escape character } & \textit{Use} \\ \hline
\backslash\text{b} & \text{1 character backward}\\ \hline
\backslash\text{n} & \text{new line}\\ \hline
\backslash\text{t} & \text{tabstop}\\ \hline
\backslash\texttt{'} & \text{single quote}\\ \hline
\backslash\texttt{"} & \text{double quote}\\ \hline
\backslash\backslash & \text{slash }\backslash
\end{array}\]